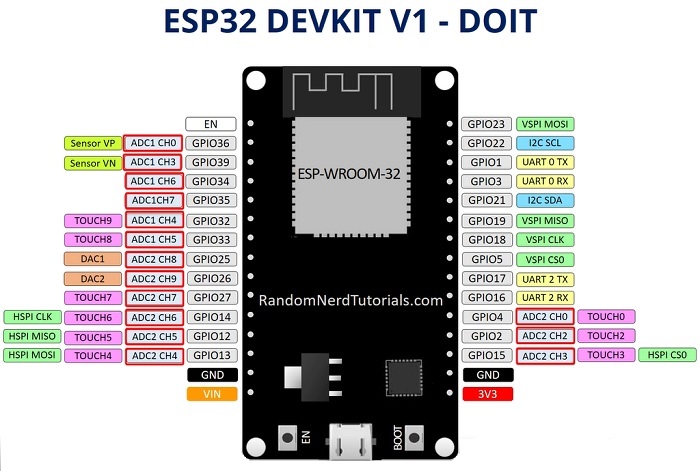
How to Use ADC on an ESP32
When it comes to working with microcontrollers like the ESP32, one of the key functionalities that you may need to use is the Analog-to-Digital Converter (ADC). The ADC on the ESP32 allows you to read analog voltage levels and convert them to digital values that can be used in your projects. In this article, we will walk you through how to use the ADC on an ESP32 board.
What is ADC?
ADC stands for Analog-to-Digital Converter, which is a device or circuit that converts a continuous analog signal into a discrete digital representation. In the case of the ESP32, the ADC allows you to read analog voltage levels and convert them into digital values that can be used by the microcontroller.
Using the ADC on an ESP32
Now that we understand what ADC is, let’s dive into how you can use the ADC on an ESP32 board. The ESP32 has a 12-bit ADC, which means it can represent analog values between 0 and 4095. Here are the steps to read analog values using the ADC on an ESP32:
- Include the ADC library:
- To use the ADC on an ESP32, you will need to include the ADC library. You can do this by including the following line at the beginning of your sketch:
#include <ADC.h>
- Initialize the ADC:
- Next, you will need to initialize the ADC by calling the
adc_begin()
function in your setup():
adc_begin();
- Read analog values:
- You can now read analog values using the
adc_read()
function. This function returns a 12-bit digital value representing the analog voltage level:
int value = adc_read();
- Convert the digital value to voltage:
- Finally, you can convert the digital value to a voltage level using the following formula:
voltage = value * 3.3 / 4095;
With these steps, you can now use the ADC on an ESP32 to read analog voltage levels and convert them to digital values that can be used in your projects. Whether you are working on a sensor-based project or need to measure analog signals, the ADC on the ESP32 can be a valuable tool in your toolkit.
Conclusion
In conclusion, the ADC on an ESP32 allows you to read analog voltage levels and convert them to digital values that can be used in your projects. By following the steps outlined in this article, you can easily start using the ADC on your ESP32 board. So go ahead, experiment with the ADC, and unlock new possibilities for your projects!
Was this helpful?
0 / 0