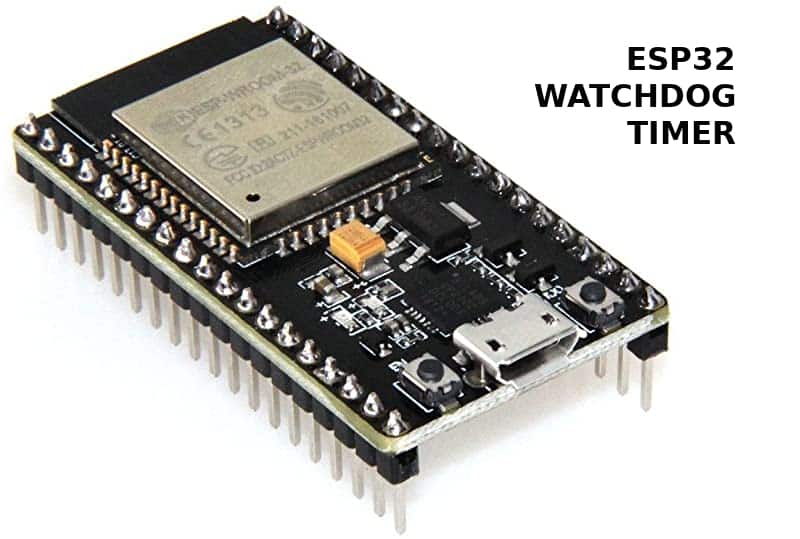
How to use a watchdog timer on ESP32
If you’re working with an ESP32 microcontroller, you may have heard about the watchdog timer but may not be entirely sure how to use it. In this article, we’ll break down what a watchdog timer is, why it’s important, and how you can effectively use it in your ESP32 projects. Let’s dive in!
What is a watchdog timer?
A watchdog timer is a hardware timer that is used to detect and recover from software anomalies. It’s an essential component in many microcontrollers to ensure system stability and prevent it from getting stuck in an unintended state. If the software fails to ‘kick’ the watchdog timer within a specified timeframe, the watchdog timer will reset the system to recover from the anomaly.
Why is a watchdog timer important?
Having a watchdog timer is crucial in embedded systems where reliability is paramount. It provides a safety net against software glitches, crashes, and other unforeseen issues that may arise during operation. By using a watchdog timer, you can improve the overall robustness and reliability of your ESP32 projects.
How to use a watchdog timer on ESP32
Using a watchdog timer on an ESP32 is relatively straightforward. The ESP32 SDK provides a built-in watchdog timer API that you can leverage in your projects. Here’s a step-by-step guide on how to use a watchdog timer on ESP32:
Step 1: Include the watchdog timer library
Before you can use the watchdog timer in your ESP32 project, you need to include the watchdog timer library. This library provides the necessary functions to interact with the watchdog timer hardware. You can include the library by adding the following line of code at the beginning of your sketch:
#include "esp_task_wdt.h"
Step 2: Set up the watchdog timer
Next, you need to set up the watchdog timer parameters. You can do this by calling the esp_task_wdt_init()
function and passing in the desired timeout value in milliseconds. Here’s an example of how you can set up the watchdog timer with a timeout of 5000 milliseconds:
esp_task_wdt_init(5000, true);
Step 3: Kick the watchdog timer
To prevent the watchdog timer from resetting your system, you need to periodically ‘kick’ the watchdog timer by calling the esp_task_wdt_reset()
function. You should do this at critical points in your code where you want to ensure that your system is running smoothly. Here’s an example of how you can kick the watchdog timer:
esp_task_wdt_reset();
Step 4: Handle watchdog timer resets
If the watchdog timer expires and resets your system, you can handle this event by setting up a watchdog timer task callback function. This function will be called when the watchdog timer triggers a reset. You can define this callback function using the following syntax:
void IRAM_ATTR task_wdt_isr_user_cb(void)
{
// Your code here
}
By implementing this callback function, you can perform any necessary cleanup or recovery actions when the watchdog timer resets your system.
Conclusion
Using a watchdog timer on ESP32 is a powerful way to enhance the reliability and stability of your projects. By following the steps outlined in this article, you can effectively integrate a watchdog timer into your ESP32 applications and ensure that your system remains responsive and robust. Happy coding!
Was this helpful?
0 / 0