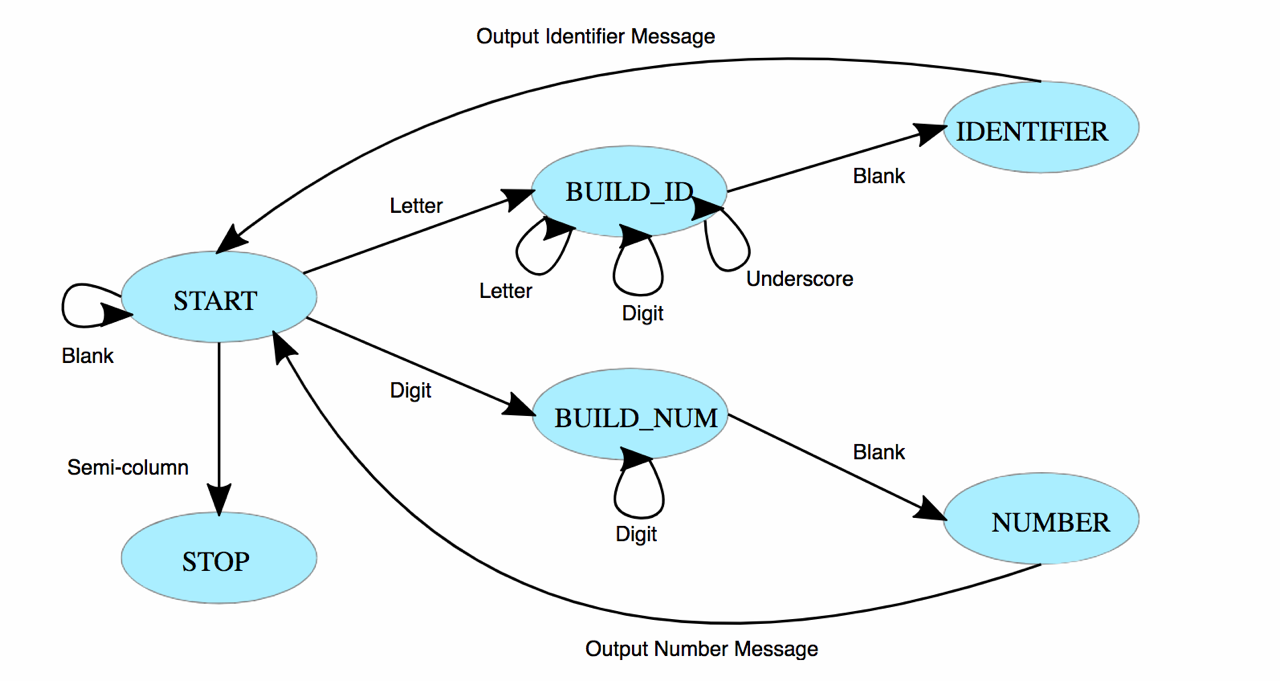
How to Implement a Simple Finite State Machine in C
If you’re looking to add more structure to your C programming projects, implementing a Finite State Machine (FSM) could be a great solution. FSMs are a powerful way to organize your code and handle complex decision-making processes in a streamlined manner. In this article, we’ll walk you through the basics of setting up a simple FSM in C.
Understanding Finite State Machines
Before we dive into the implementation details, let’s first understand what a Finite State Machine is. At its core, an FSM consists of a set of states, transitions between those states, and actions that are triggered by those transitions.
Each state represents a specific condition or situation in your program, and transitions define how your program moves from one state to another based on certain conditions. Actions, on the other hand, are functions or operations that are executed when a transition occurs.
Implementing a Simple FSM in C
Now, let’s get into the nitty-gritty of implementing a basic FSM in C. We’ll break down the process into a few key steps:
- Define your states: Start by defining all the possible states that your program can be in. This could include states like “Idle,” “Running,” or “Error.”
- Define your transitions: Next, define the conditions that cause your program to move from one state to another. For example, a transition from “Idle” to “Running” could be triggered by a user input.
- Implement your actions: Finally, implement the actions that should be taken when a transition occurs. This could involve performing certain computations, changing variables, or triggering other functions.
With these steps in mind, let’s put together a simple FSM example in C:
#include
// Define the states
typedef enum {
Idle,
Running,
Error
} State;
// Define the transitions
typedef struct {
State currentState;
State nextState;
int triggerCondition;
} Transition;
// Define the actions
void startRunning() {
printf("Starting the program...\n");
}
void handleError() {
printf("An error has occurred.\n");
}
// Main function
int main() {
State currentState = Idle;
Transition transitions[] = {
{Idle, Running, 1},
{Running, Error, 0}
};
// Main loop
while (1) {
for (int i = 0; i < sizeof(transitions) / sizeof(transitions[0]); i++) {
if (currentState == transitions[i].currentState && transitions[i].triggerCondition) {
currentState = transitions[i].nextState;
// Perform actions based on transitions
switch (currentState) {
case Running:
startRunning();
break;
case Error:
handleError();
break;
default:
break;
}
}
}
}
return 0;
}
In this example, we have defined three states (Idle, Running, Error), two transitions, and two actions that are triggered by those transitions. The program continuously loops through the defined transitions and performs the necessary actions based on the current state.
Conclusion
Implementing a Finite State Machine in C can be a powerful tool for simplifying your code and making it more robust. By breaking down your program into states, transitions, and actions, you can create a clear and organized structure that is easier to understand and maintain.
We hope this article has given you a good starting point for implementing your own FSM in C. Feel free to experiment with different states, transitions, and actions to suit your specific project requirements. Happy coding!
Was this helpful?
0 / 0