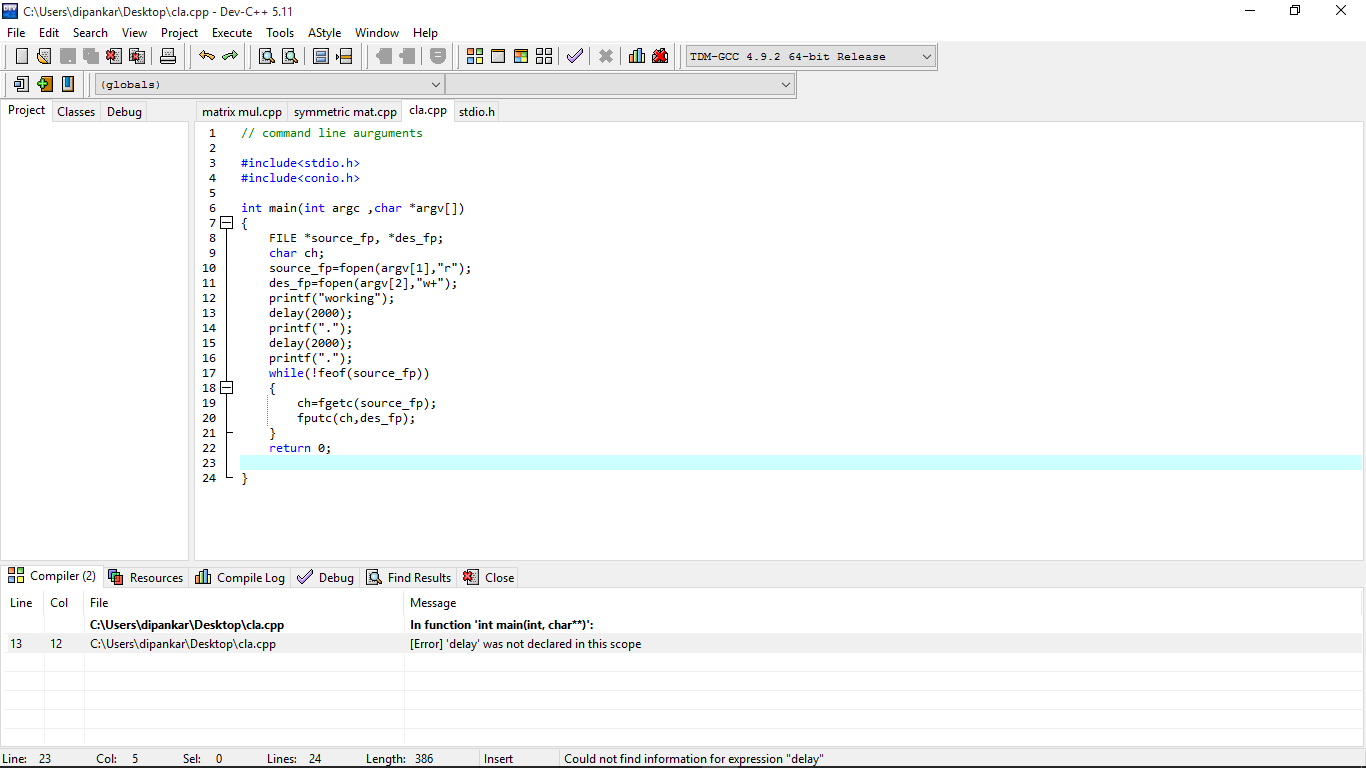
Best Way to Create a Delay Function in C
When programming in C, there may be occasions where you need to create a delay in your code. Whether you are waiting for user input or simulating a real-world scenario, implementing a delay function can be useful. In this article, we will explore the best ways to create a delay function in C.
Method 1: Using sleep() Function
One of the simplest ways to create a delay in C is by using the sleep()
function. This function pauses the program execution for a specified number of seconds. Here is an example of how to use the sleep()
function:
#include
#include
int main() {
printf("Hello, world!\n");
sleep(5); // Delay program execution for 5 seconds
printf("Delayed message\n");
return 0;
}
In the code snippet above, the sleep(5)
function call pauses the program execution for 5 seconds before printing the “Delayed message” onto the console. While this method is simple and easy to implement, it has one major drawback – it blocks the entire program during the delay.
Method 2: Using Busy Wait Loop
Another way to create a delay in C is by using a busy wait loop. This method involves continuously checking the elapsed time until the desired delay is achieved. Here is an example code snippet demonstrating how to use a busy wait loop:
#include
#include
void delay(int seconds) {
clock_t start_time = clock();
while ((clock() - start_time) / CLOCKS_PER_SEC < seconds);
}
int main() {
printf("Hello, world!\n");
delay(5); // Delay program execution for 5 seconds
printf("Delayed message\n");
return 0;
}
In the code above, the delay()
function uses a busy wait loop to pause the program execution for the specified number of seconds. While this method does not block the entire program, it consumes CPU resources and may not be suitable for long delays.
Method 3: Using Timer and Signal Handling
For more precise and efficient delay handling, you can use timers and signal handling in C. By setting up a timer with the desired delay and handling the timer signal, you can achieve accurate delays without blocking program execution. Here is a sample code snippet showcasing this method:
#include
#include
#include
void handler(int signal) {
// Do nothing on signal interrupt
}
void delay(int seconds) {
struct sigaction sa;
sa.sa_handler = &handler;
if (sigaction(SIGALRM, &sa, NULL) == -1) {
perror("Error setting up signal handler");
return;
}
alarm(seconds);
pause(); // Wait until signal interrupt
}
int main() {
printf("Hello, world!\n");
delay(5); // Delay program execution for 5 seconds
printf("Delayed message\n");
return 0;
}
In the code snippet above, the delay()
function uses a timer and signal handling to create a precise delay without blocking the program execution. By setting up a signal handler for the alarm signal, the program pauses until the timer expires, achieving the desired delay.
When creating a delay function in C, consider the specific requirements of your application and choose the method that best suits your needs. Whether you opt for a simple sleep()
function, a busy wait loop, or timer and signal handling, be mindful of the trade-offs in terms of program responsiveness and resource utilization.
Experiment with these methods and explore other techniques to create delays in your C programs. By understanding the different approaches to implementing delays, you can optimize your code for better performance and efficiency.
Was this helpful?
0 / 0