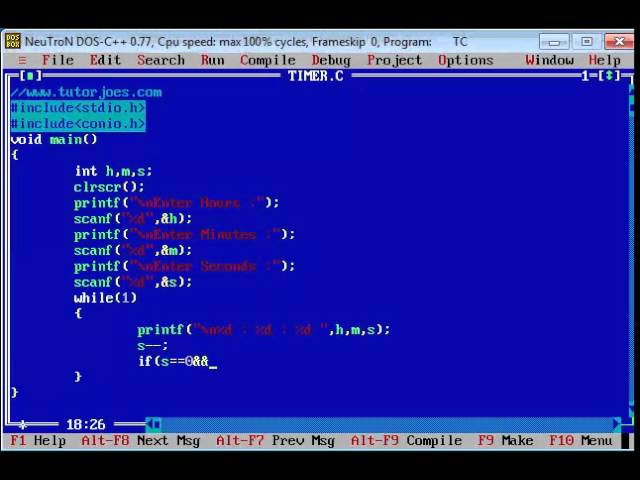
Best Way to Implement a Software Timer in C
When it comes to implementing a software timer in C, there are a few key considerations to keep in mind. Software timers are essential for executing tasks at specific intervals, and knowing how to properly implement them can greatly improve the efficiency and accuracy of your code.
In this article, we will discuss the best way to implement a software timer in C, including some tips and tricks to help you get started.
What is a Software Timer?
A software timer is a mechanism used in embedded systems programming to execute tasks at specific intervals. This is crucial for tasks that need to be performed periodically, such as updating an LCD display or reading sensor data.
Software timers are typically implemented using either hardware timers or software counters. Hardware timers are built into the microcontroller and can be used to trigger an interrupt at regular intervals. Software counters, on the other hand, are variables that are updated periodically and checked to see if a certain amount of time has elapsed.
Implementing a Software Timer in C
There are several ways to implement a software timer in C, but one of the most common methods is to use a timer interrupt. A timer interrupt is triggered by a hardware timer at regular intervals and can be used to execute a specific function. Here’s a simple example of how you can implement a software timer using timer interrupts:
#include <avr/interrupt.h>
#include <avr/io.h>
volatile unsigned long milliseconds = 0;
ISR(TIMER0_COMPA_vect)
{
milliseconds++;
}
In this example, we are using an AVR microcontroller and the Timer/Counter 0 to generate a timer interrupt every millisecond. The ISR (Interrupt Service Routine) increments a volatile variable milliseconds
every time the interrupt is triggered.
Once you have set up the timer interrupt, you can use the milliseconds
variable to keep track of time in your application. For example, you can use it to trigger a specific function every second or to measure the elapsed time between two events.
Tips for Implementing Software Timers
- Choose an appropriate timer resolution based on your application’s requirements. For example, if you need to execute a task every second, you should set the timer interval to 1000 milliseconds.
- Make sure to keep the timer interrupt routine as short and efficient as possible to minimize any impact on the rest of your code.
- Use volatile variables to store timer values that are updated within an interrupt routine to prevent compiler optimization issues.
- Consider using a software counter if hardware timers are not available or if you need more flexibility in setting timer intervals.
By following these tips and best practices, you can effectively implement a software timer in C and improve the performance of your embedded systems applications. Software timers are essential for managing time-sensitive tasks and can help you create more reliable and efficient code.
So, the next time you’re working on an embedded systems project that requires precise timing control, be sure to consider implementing a software timer in C using timer interrupts or software counters for optimal results.
Was this helpful?
0 / 0