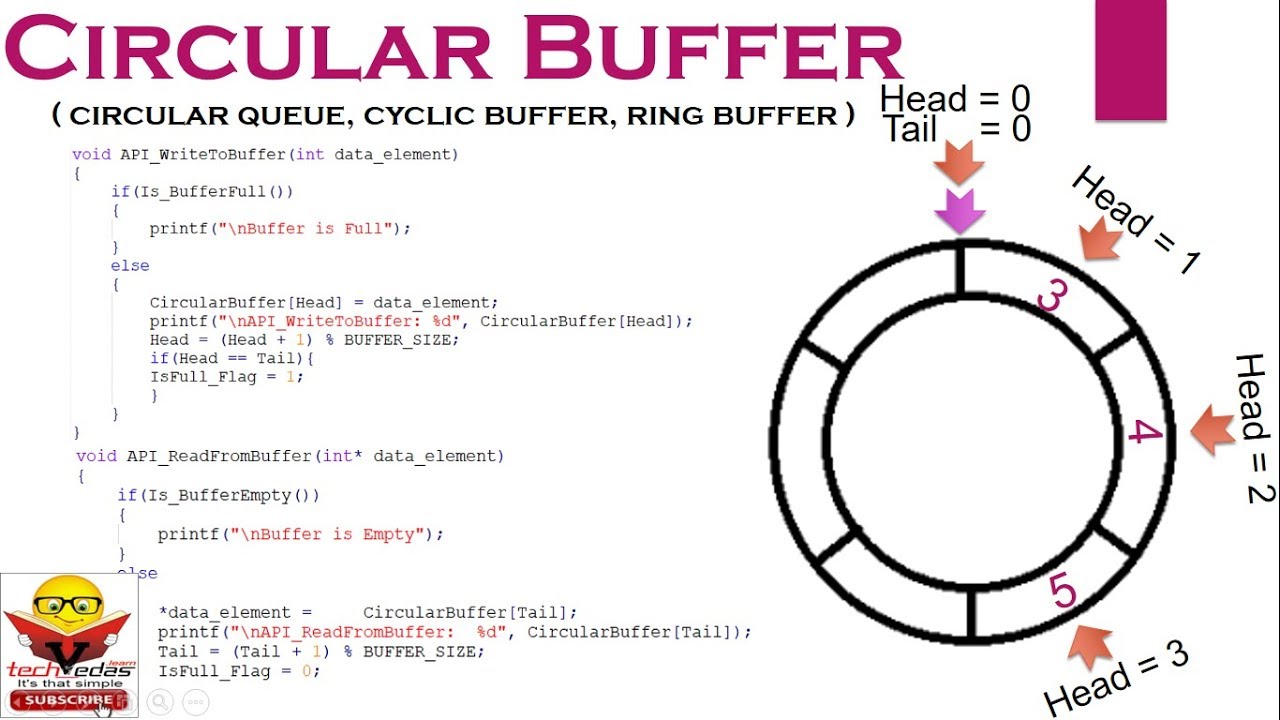
How to implement a circular buffer in embedded C?
When working with embedded systems, efficient memory management is crucial for optimizing performance and functionality. One common data structure used in embedded C programming is a circular buffer. A circular buffer is a fixed-size data structure that follows the FIFO (First In, First Out) principle, making it ideal for implementing data buffers in embedded systems.
In this article, we will discuss how to implement a circular buffer in embedded C, along with some best practices and tips for efficient usage.
What is a Circular Buffer?
A circular buffer, also known as a ring buffer or cyclic buffer, is a data structure that uses a single, fixed-size buffer as if it were connected end-to-end. When data is added to the buffer, it is written to the current position, and the position is then incremented. Once the buffer is full, new data overwrites the oldest data in a circular manner. This allows for efficient storage and retrieval of data without the need for complex memory management.
Implementing a Circular Buffer in Embedded C
Implementing a circular buffer in embedded C involves creating a structure to hold the buffer data, as well as functions to initialize, read from, and write to the buffer. Here is a basic example of a circular buffer implementation:
// Define the circular buffer structure
typedef struct {
uint8_t buffer[SIZE];
int head;
int tail;
} CircularBuffer;
Once the structure is defined, you can create functions to initialize the buffer, write data to the buffer, and read data from the buffer. Here is an example of how to initialize the circular buffer:
// Initialize the circular buffer
void initialize_buffer(CircularBuffer* cbuf) {
cbuf->head = 0;
cbuf->tail = 0;
}
Best Practices for Circular Buffers
- Always check if the buffer is empty before reading data.
- Ensure proper synchronization when reading and writing to the buffer in a multi-threaded environment.
- Avoid resizing the buffer dynamically, as it can lead to data corruption and performance issues.
- Use circular buffers for applications where data is produced and consumed at different rates.
Conclusion
Implementing a circular buffer in embedded C can help improve memory management and optimize data storage in embedded systems. By following best practices and understanding the principles of circular buffers, you can create efficient and reliable data buffers for your embedded applications.
Thank you for reading this article, and we hope you found it helpful for implementing circular buffers in embedded C!
Was this helpful?
0 / 0