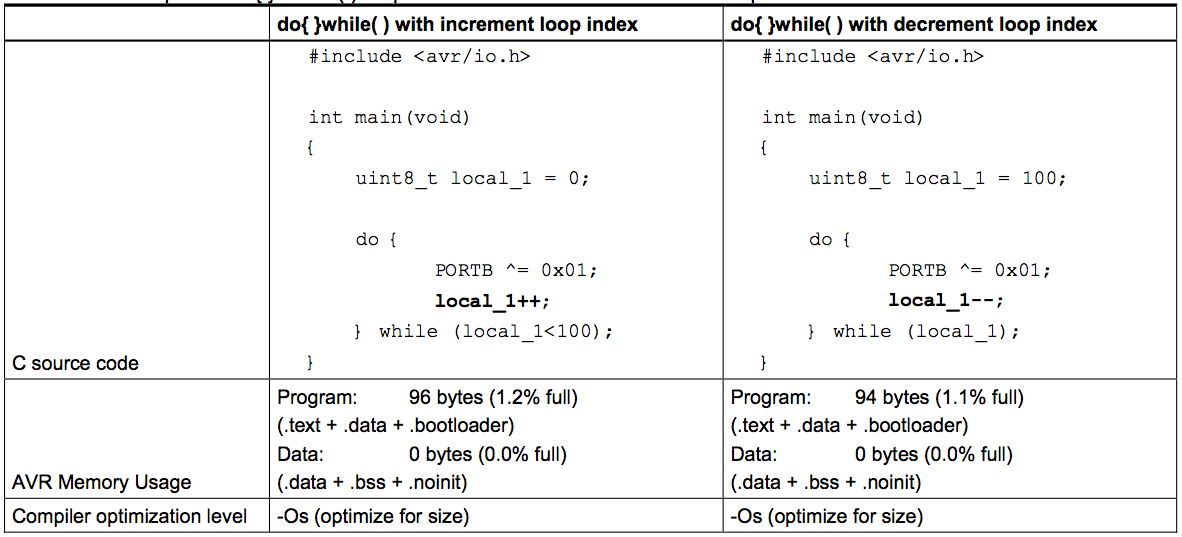
How to Write Efficient C Code for Microcontrollers
Microcontrollers are small computing devices used in a wide range of applications, from embedded systems to IoT devices. Writing efficient C code for microcontrollers is essential to maximizing performance and minimizing resource usage. In this article, we’ll explore some tips and best practices for writing efficient C code for microcontrollers.
1. Choose the Right Data Types
Choosing the right data types can have a significant impact on the efficiency of your code. Use data types that are appropriate for the range of values you need to store. For example, if you only need to store values from 0 to 255, using an unsigned char instead of an int can save memory and improve performance.
2. Avoid Unnecessary Variables
Avoid declaring unnecessary variables in your code. Each variable takes up memory, so reducing the number of variables can help conserve memory and improve performance. Make sure to only declare variables that are essential for the functionality of your code.
3. Use Bitwise Operations
Bitwise operations can be more efficient than arithmetic operations in many cases. For example, using bitwise << and >> operators can be faster than multiplying or dividing by powers of 2. Be sure to use bitwise operations where appropriate to improve the efficiency of your code.
4. Optimize Loops
Loops are a common programming construct, but they can also be a source of inefficiency in your code. Make sure to optimize loops by minimizing the number of iterations and avoiding unnecessary calculations within the loop. Consider using loop unrolling or loop fusion techniques to improve loop performance.
5. Minimize Function Calls
Function calls can introduce overhead in your code, especially on resource-constrained microcontrollers. Minimize the number of function calls by combining small functions into larger ones or by using inline functions where possible. This can help reduce the overhead associated with function calls and improve the efficiency of your code.
6. Use Static Variables
Static variables retain their value between function calls, which can be useful for storing information that needs to persist across function invocations. Using static variables can reduce the need for global variables and improve code readability. Just be mindful of potential side effects when using static variables in your code.
7. Enable Compiler Optimizations
Most C compilers come with optimization flags that can help improve the efficiency of your code. Enable compiler optimizations to allow the compiler to perform various transformations on your code to make it faster and more efficient. Experiment with different optimization levels to find the best performance for your microcontroller project.
8. Test and Iterate
Finally, be sure to test your code on the target microcontroller platform and iterate on your design. Use profiling tools to identify bottlenecks in your code and make optimizations where necessary. By testing and iterating on your code, you can ensure that it runs efficiently on your microcontroller and meets the performance requirements of your project.
By following these tips and best practices, you can write efficient C code for microcontrollers that maximizes performance and minimizes resource usage. Remember to consider the constraints of your microcontroller platform and make optimizations accordingly. Happy coding!
Was this helpful?
0 / 0