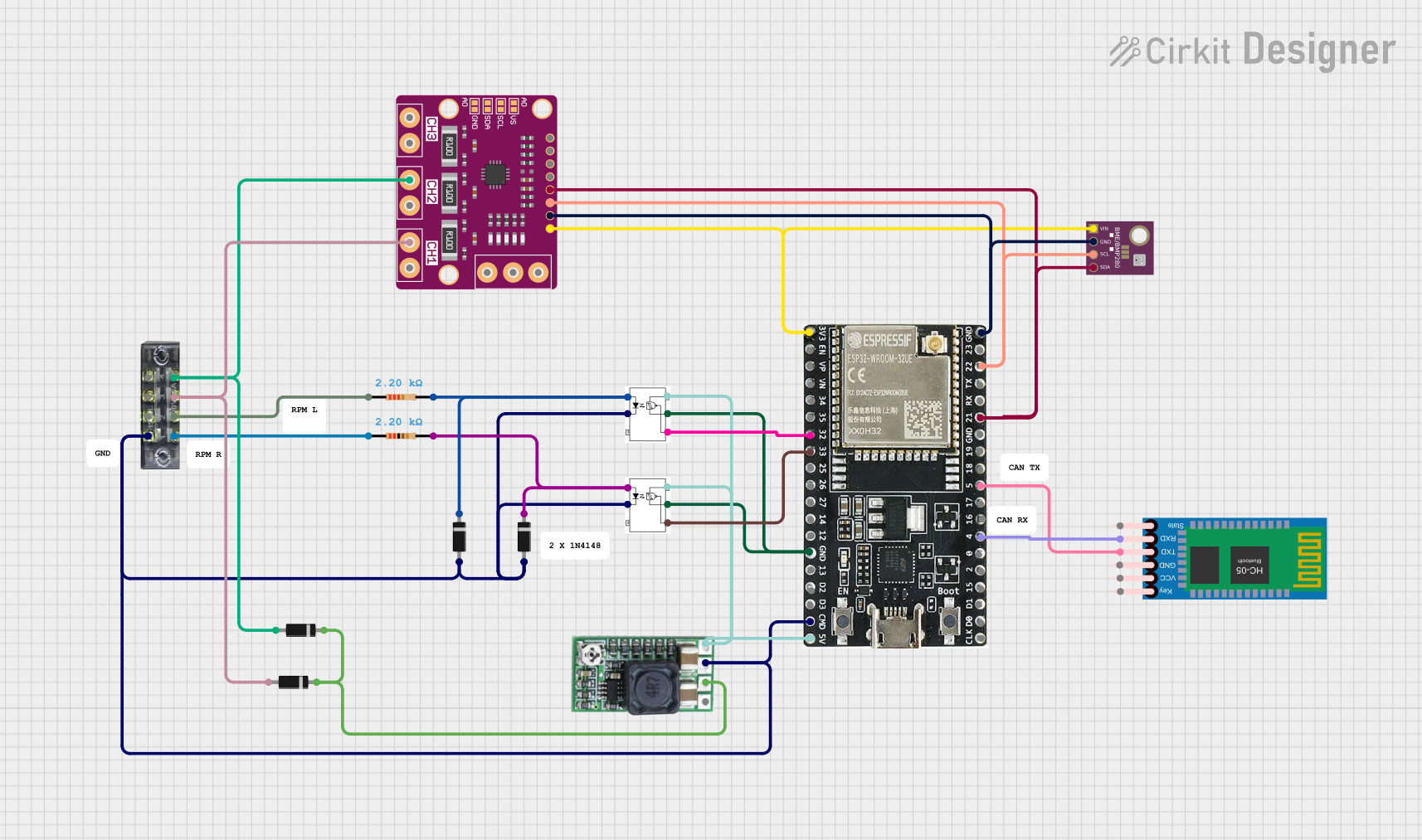
How to Use an INA3221 Power Monitor with ESP32
If you’re looking to monitor power usage in your ESP32 projects, using an INA3221 power monitor can be a great solution. The INA3221 is a current shunt and power monitor that can measure three channels simultaneously, making it ideal for monitoring multiple power sources in your ESP32 setup.
In this guide, we’ll walk you through the process of connecting and using an INA3221 power monitor with an ESP32 microcontroller. By the end of this tutorial, you’ll be able to monitor power consumption in your projects with ease.
Materials Needed:
- ESP32 development board
- INA3221 power monitor module
- Jumper wires
- USB cable for programming
- Computer with Arduino IDE installed
Step 1: Wiring
Start by wiring the ESP32 and the INA3221 power monitor module. Connect the following pins:
- ESP32 3.3V -> INA3221 VIN
- ESP32 GND -> INA3221 GND
- ESP32 SDA -> INA3221 SDA
- ESP32 SCL -> INA3221 SCL
Step 2: Installing Libraries
Next, open the Arduino IDE on your computer and install the following libraries:
- Adafruit BusIO
- Adafruit INA3221
You can install these libraries by going to Sketch > Include Library > Manage Libraries
and searching for the libraries by name.
Step 3: Coding
Now, it’s time to write the code for your ESP32 to read data from the INA3221 power monitor. You can use the following example code to get started:
#include
#include
Adafruit_INA3221 ina3221 = Adafruit_INA3221();
void setup() {
Serial.begin(115200);
ina3221.begin();
}
void loop() {
Serial.print("Bus Voltage: ");
Serial.print(ina3221.getBusVoltage_V(1));
Serial.println(" V");
Serial.print("Shunt Voltage: ");
Serial.print(ina3221.getShuntVoltage_mV(1));
Serial.println(" mV");
Serial.print("Current: ");
Serial.print(ina3221.getCurrent_mA(1));
Serial.println(" mA");
Serial.println();
delay(1000);
}
Step 4: Monitoring Power Consumption
Upload the code to your ESP32 and open the Serial Monitor in the Arduino IDE. You should see the bus voltage, shunt voltage, and current consumption values being displayed. This data will help you monitor the power usage of your ESP32 project.
By following these steps, you’ll be able to easily use an INA3221 power monitor with your ESP32. This setup is perfect for monitoring power consumption in your projects and ensuring optimal performance.
Experiment with different INA3221 configurations and code modifications to tailor the power monitoring setup to your specific needs. Have fun monitoring your ESP32 power consumption and optimizing your projects for efficiency!
Was this helpful?
0 / 0