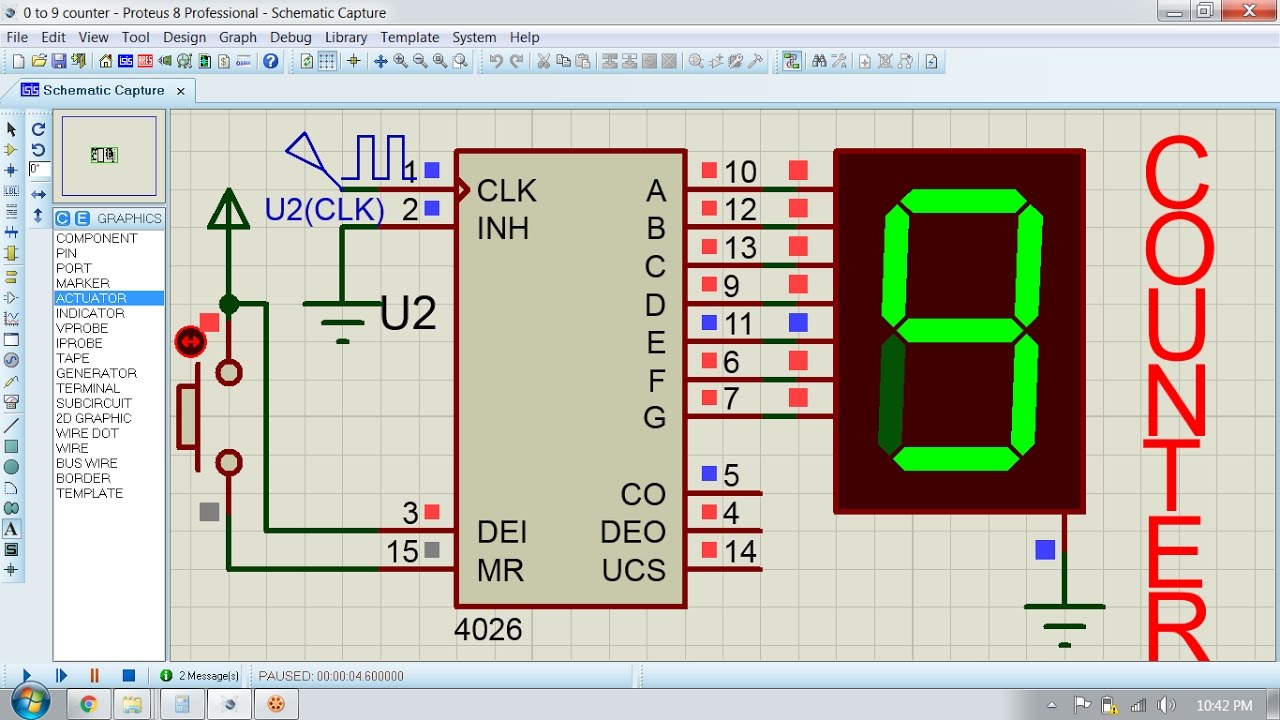
How to Create a Simple Digital Counter
If you ever wanted to create a simple digital counter for a website or an app, you’ve come to the right place. In this tutorial, we’ll show you how to set up a basic digital counter using HTML, CSS, and JavaScript. It’s a fun and beginner-friendly project that you can customize to suit your needs. Let’s get started!
Getting Started
First, create a new HTML file and name it index.html
. This will be the main file where all the magic happens. Make sure you have a text editor like Visual Studio Code or Sublime Text to write and edit your code.
Next, let’s set up the basic structure of our HTML file. Copy and paste the following code into your index.html
file:
```html
Digital Counter
0
```
Save your file and create two new files called styles.css
and script.js
in the same directory as your index.html
file. We’ll use these files to style our counter and add functionality to it.
Styling the Counter
Open your styles.css
file and add the following CSS code. This code will style our counter and buttons:
```css
body {
font-family: Arial, sans-serif;
text-align: center;
}
.counter {
margin: 50px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
h1 {
font-size: 3em;
}
button {
margin: 0 10px;
padding: 10px 20px;
font-size: 1.5em;
}
```
Adding Functionality
Now, let’s add some JavaScript to make our counter work. Open your script.js
file and paste the following code:
```javascript
let count = 0;
const counter = document.querySelector('h1');
function increment() {
count++;
counter.innerText = count;
}
function decrement() {
if (count > 0) {
count--;
counter.innerText = count;
}
}
```
This JavaScript code initializes a count variable to 0 and selects the <h1>
element where our count will be displayed. The increment()
function increases the count by 1 when the + button is clicked, and the decrement()
function decreases the count by 1 when the – button is clicked.
Final Touches
That’s it! You’ve successfully created a simple digital counter using HTML, CSS, and JavaScript. Feel free to customize the styles or add new features to make it your own. This project is a great starting point for anyone looking to learn web development basics. Have fun coding!
Was this helpful?
0 / 0